We will in this article show you a couple of great examples to get you started with Angular signals. We’ll also explain the difference between Angular signals and other reactive libraries.
Are you ready to make your Angular applications more event-driven without adding a lot of complex operators and logic? Keep scrolling to find out how
What Are Angular Signals?
Signals are a new way of handling asynchronous event streams in Angular. The dependency to the event stream is defined upon declaration and you can easily add callback functions to it.
Multiple types of signals exist in Angular, where the commonly used ones are the writable signals and computed signals.
Here’s how you define a signal in Angular, in this case, a writeable signal:
mySignal = signal(0); //This declares a signal with an initial value of zero
Ensure you are running an Angular version >= 16 since this functionality was introduced in Angular 16.
To test this out, we can add a computed signal which will hold the doubled value of mySignal
:
doubleValue = computed(() => this.mySignal() * 2);
And then, to display the results, we can add the following HTML:
<button (click)="mySignal.set(mySignal() + 1)">Increase Value</button>
<br />
mySignal = {{ mySignal() }} | doubleValue = {{ doubleValue() }}
We can now run our application and increase the value of our signal by clicking on the button. When the value of our signal changes, the computed signal will multiply the value by 2. Here’s what it looks like:
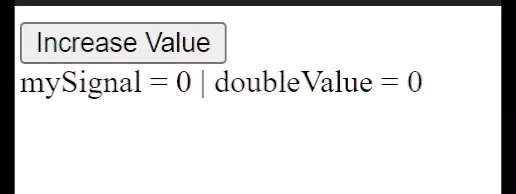
That’s one of the easiest examples of Angular signals. Next up, we’ll take a look at an example where signals simplify our development process and improve the user experience of our Angular applications.
Angular Signals Example
We’ll now go through an example of when you can use signals in Angular to easily handle event and data streams.
Shopping Cart Using Angular Signals
Let’s create a simple application that enables the user to add random items to a shopping cart and display a list of the items and the total price.
In this example, we’ll be using a writeable signal and a computed signal.
I created a new component for this example:
ng g c shopping-cart
Next up, we’ll define our signal containing all items and the computed signal which calculates the total price:
signal: WritableSignal<Item[]> = signal([]);
totalCost: Signal<Number> = computed(() => {
return this.signal().reduce((sum, item) => sum + item.price, 0);
});
The model, Item
, looks like this:
export interface Item {
name: string;
price: number;
}
Also add functions for removing an item, adding an item, and removing all items:
removeItem(item: Item) {
this.signal.set(this.signal().filter((i) => i !== item));
}
removeAllItems() {
this.signal.set([]);
}
addItem() {
this.signal().push({
name: faker.commerce.productName(),
price: faker.number.int({ max: 500 }),
});
this.signal.set(this.signal());
}
Now we just need some basic HTML for displaying our shopping cart application:
<h1>My Shopping Cart</h1>
<button (click)="addItem()">Add</button> |
<button (click)="removeAllItems()">Remove all items</button>
<hr />
<ul>
<li *ngFor="let item of signal()">
{{ item.name }} - ${{ item.price }}
<button (click)="removeItem(item)">Remove</button>
</li>
</ul>
<b>Total Price: ${{ totalCost() }}</b>
Now, run your application and enjoy the results!
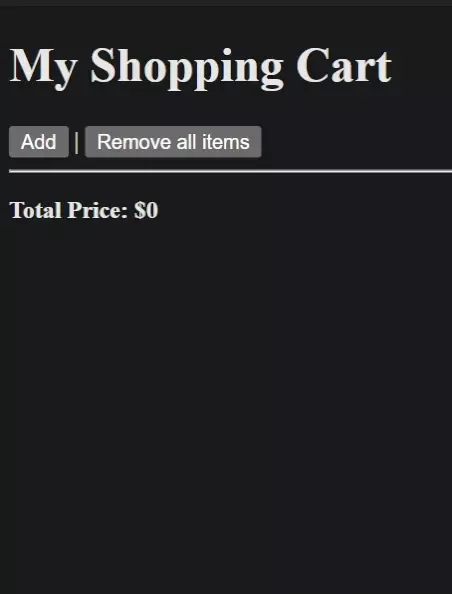
Signals vs Other Reactive Methods
Signals was introduced in Angular 16 but the concept of reactivity and event-driven development have been around for a long time.
Other web frameworks like React, Vue, and Svelte already have this kind of functionality built-in.
Multiple external libraries with a focus on event-driven development exist today, with the biggest and most popular being the RxJs library.
Angular Signals is just a nice feature released for Angular. It will probably not replace the other reactive libraries which exist today.
Conclusion
In conclusion, signals are a new and easy way of handling asynchronous streams in Angular, just like already existing libraries like RxJs.
It may sound like signals is a replacement to other event-driven solutions but we are certain that the other tools and libraries will still exist and be used. Angular Signals will most probably be used in conjunction with the other existing tools and libraries.
Hope you enjoyed reading this article about Angular Signals and that you found it helpful.
Did you know that we have a collection of some great tutorials on Angular Material? If you’re interested in learning everything about Angular Material, check out the list of tutorials here!