When working with JavaScript, it is important to understand the differences between the equality operator ==
and the strict equality operator ===
. While both operators are used to compare values, they have some key differences.
In this article, we’ll explain the two operators and what they do and then compare them against each other.
What Is The Equality (==) Operator?
The ==
operator is known as the equality operator, and it will compare two values for equality after converting them to the same type. This means that, for example, the number 1 will be considered equal to the string “1”. This can sometimes lead to unexpected results, as the type conversion can change the meaning of the values being compared.
Here is an example of using the equality operator ==
in JavaScript:
var x = 1;
var y = "1";
if (x == y) {
console.log("x and y are equal");
} else {
console.log("x and y are not equal");
}
Because the ==
operator converts the y
variable (which is a string) to a number before comparing it to x
, the if statement will be evaluated as true in this case. This means that even though the two values are of different types, they will be treated as equal.
As a result, the console.log
in the if
block will be executed and the message "x and y are equal"
will be printed to the console.
What Is The Strict Equality (===) Operator?
On the other hand, the ===
operator is known as the strict equality operator, and it will only consider two values equal if they are of the same type and have the same value. Using the same example above, the number 1 will not be considered equal to the string “1” when used with the strict equality operator.
In the same example as the earlier but using the strict equality operator ===
instead of the equality operator:
var x = 1;
var y = "1";
if (x === y) {
console.log("x and y are equal");
} else {
console.log("x and y are not equal");
}
In this case, the ===
operator will not perform any type of conversion before comparing the values of x
and y
, hence the if statement will be evaluated as false. The values are not strictly equal because x
is a number and y
is a string.
As a result, the console.log
statement in the else
block will be executed and the message “x and y are not equal” will be printed to the console.
When Is It Important To Use The Strict Equality Operator In JavaScript?
Below are two great tables, the equality table (to the left) and the strict equality table (to the right):

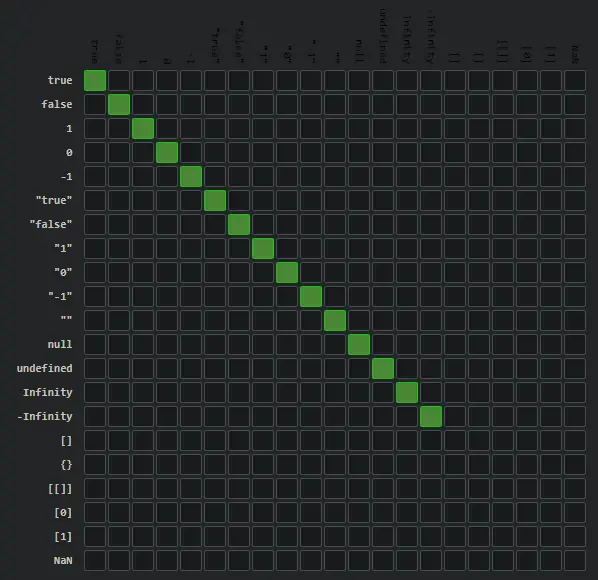
The main reason why you should use the strict equality operator in JavaScript is that it can help prevent bugs in your code. By ensuring that values are of the same type before comparing them, you can avoid unexpected behavior that might otherwise be caused by type conversion.
Another advantage of using the strict equality operator is that it can make your code more readable. Since the ===
operator only considers two values equal if they are of the same type, it can make it clear to other developers reading your code what types of values you are expecting. This can make it easier for others to understand your code and identify potential bugs.
I really like the following quote from dorey.github.io:
When using two equals signs for JavaScript equality testing, some funky conversions take place.
https://dorey.github.io/JavaScript-Equality-Table/
Moral Of The Story: Always use 3 equals unless you have a good reason to use 2.
Conclusion
In summary, it is generally considered best practice to use the strict equality operator ===
instead of the equality operator ==
in JavaScript. You should therefore not use the equality operator unless you have a very good reason for using it.
I hope this article answered your question and/or that you enjoyed reading it.
Whether you are a beginner looking to learn the fundamentals of web development or an experienced developer looking to expand your knowledge, these articles will provide valuable information and insights into this exciting field. If you are more interested in Javascript, you should check out our other articles here