This tutorial will show you how easy it is to create an API using Nodejs and Express. We’ll create one GET endpoint in this example, which you’ll be able to call from other applications through the HTTP protocol.
Setting Up Our Nodejs+Express Project
Start by creating a new directory and initialize npm
inside it:
mkdir my-first-api && cd my-first-api && npm init
You can use the default config for npm, just press enter on each option until the setup is complete.
Continue by installing express
to our project and nodemon
as a dev dependency:
npm i express && npm i nodemon --save-dev
nodemon is a small npm package used for automatically restarting a running server when any file changes
As a last thing before we start creating our API, add a new npm
command for starting the dev server:
{
"name": "my-first-api",
"version": "1.0.0",
"description": "",
"main": "index.js",
"scripts": {
"test": "echo \"Error: no test specified\" && exit 1",
"dev": "nodemon index.js"
},
"author": "",
"license": "ISC",
"dependencies": {
"express": "^4.18.2"
},
"devDependencies": {
"nodemon": "^3.0.3"
}
}
package.jsonand, create the index.js
file:
touch index.js
if you now run the command, npm run dev
, you should see a message in the terminal telling you that the nodemon
server is running:
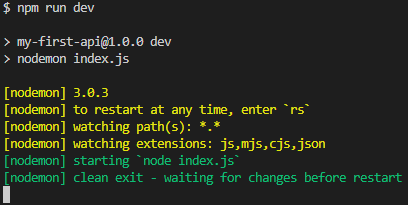
Configuring The Express Server
We managed to get the nodemon
server running but that’s useless unless we don’t configure our express server and expose it. So, that’s what we’re going to do now.
Start by importing all required dependencies at the top of our index.js
file and then exposing port 8000 on our API:
const express = require('express');
const app = express();
const port = 8000;
app.listen(port, () => {
console.log('Listening on port ' + port);
});
index.jsIf you have the nodemon
server running, you should see it restarting the server after you save the changes in index.js
:
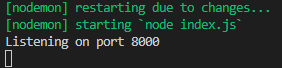
Adding Our First API Endpoint
We have our API running and exposing port 8000, what we need to do now is to add a GET endpoint to it:
const express = require('express');
const app = express();
const port = 8000;
app.get('/', (_, res) => res.send('Hello World'));
app.listen(port, () => {
console.log('Listening on port ' + port);
});
index.jsIf you save the file and have your API running, you can go to the following URL in your browser; http://localhost:8000, and you should get the following result:
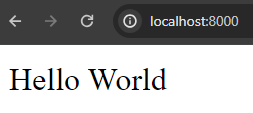
Conclusion
In just a few minutes, we managed to create a working API using Nodejs and Express. By following just a few simple steps, we learned how to set up a new Nodejs API project, how to simplify development and testing by using nodemon
, and how to add endpoints to our API.
If you’re interested in learning more about web development, then you should check out our article about Cookies and other ways of storing data on the web, or why not learn about the difference between JavaScript and TypeScript?