A slider is a common tool for selecting a predefined value or range between an interval. Angular Material provides an effortless way of adding nice-looking sliders to your Angular applications in just a few lines of code, so let’s dig into that.
This tutorial will show you how to add sliders to your Angular application using the mat slider component in Angular Material. We’ll also show you different Angular Material slider examples, how to add informative thumb labels, and how to adjust the slider sensitivity by defining the step size.
Are you ready to learn about Angular Material sliders? Then, keep on scrolling!
Creating Your First Slider Using Angular Material
It is very easy to add a slider to your Angular application using Angular Material. To display a slider with a draggable thumb, you only need 3 lines of HTML.
First, you need to make sure to import MatSliderModule
to your application:
import { MatSliderModule } from "@angular/material/slider";
...
imports: [
...
MatSliderModule,
]
app.module.tsNext, you can add the element (mat slider) to your component’s HTML:
<mat-slider>
<input matSliderThumb />
</mat-slider>
That’s all there is to it… at least if you only want to display a basic slider in your application. Here’s what it looks like:

Creating An Angular Range Slider
A basic slider for selecting a value is all fun and great but what if you want to select a range of values? Then, you’re in luck since this is supported by Angular Material and it’s just as easy as creating a basic slider.
The only difference between a range slider and a regular slider in Angular Material is the number of inputs your mat-slider
element needs to contain. If you want to create a range slider you need to have two inputs inside the mat-slider
element, matSliderStartThumb
and matSliderEndThumb
.
This will create a mat-slider with two thumbs:
<mat-slider>
<input matSliderStartThumb>
<input matSliderEndThumb>
</mat-slider>
Easy, right? And this is how the range slider works and looks like:

Add Thumb Label To Angular Material Slider
So far we’ve covered how to create a basic slider and a range slider, using Angular Material. Now, it’s time to move on to adding thumb labels to our Angular slider.
The thumb label is not shown by default. In order to show it, you need to add the discrete
property to the mat-slider
:
<mat-slider discrete>
<input matSliderThumb />
</mat-slider>
It now shows the mat slider value when you hover it:
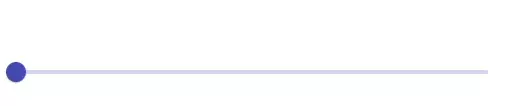
Custom Thumb Label Formatting
Sometimes, only showing the current value of a slider is not enough. In these cases, you can utilize a custom function formatting the slider’s thumb label.
The Angular Material slider component, mat-slider
, have a property called displayWith
. This property accepts all functions that take a number value as input and returns a string.
Here’s what the property definition says:
Function that will be used to format the value before it is displayed in the thumb label. Can be used to format very large number in order for them to fit into the slider thumb.
Official Angular Material Source Code
So, after understanding this documentation, let’s create our formatting function:
import { Component } from "@angular/core";
@Component({
selector: "app-slider",
templateUrl: "./slider.component.html",
styles: [],
})
export class SliderComponent {
formatThumbLabel(value: number): string {
return `${value} 👍`;
}
}
slider.component.tsNow, set the displayWith
property:
<mat-slider discrete [displayWith]="formatThumbLabel">
<input matSliderThumb />
</mat-slider>
And here’s the result:
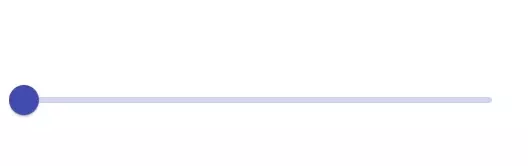
How to Adjust Slider Sensitivity (Setting Step Size)
When using the slider component from Angular Material you can easily adjust the sensitivity of it. For example, you may want to increase/decrease the value by increments of 10.
In order to change the slider sensitivity so it increases/decreases the value by increments of 10 you can set the step
property on the mat-slider
element, like this:
<mat-slider discrete [displayWith]="formatThumbLabel" step="10">
<input matSliderThumb />
</mat-slider>
This is how the slider works now:
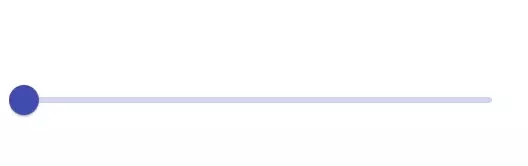
Conclusion
This tutorial goes through how to create a slider using Angular Material and showcases some examples of how to customize it according to your application’s needs.
You should now know how to add sliders to your Angular applications, how to add informative thumb labels, and how to adjust the slider sensitivity.
You can check out the Angular Material Slider API if you’re interested in digging deeper into the slider component, you can find it here!
If you want to learn more about other Angular Material components, check out our list of all our Angular Material tutorials here!