This short but informative tutorial will guide you through how to add loading spinners to Angular Material buttons. For this tutorial, we assume that you already have an Angular project with Angular Material installed that you can work on.
We’ll use one Angular component in this tutorial and all code we write will be put into that component
Adding the CSS
Let’s start by defining the styling for our spinner inside the button.
We’ll call our CSS class spinner
and we can start by adding the keyframe for rotating it 360 degrees:
@keyframes spinner {
to {transform: rotate(360deg);}
}
And then there’s some nitty-gritty styling to make the spinner look aesthetically pleasing, which you can copy and paste into the inline styling inside your component:
.spinner:before {
content: '';
box-sizing: border-box;
position: absolute;
top: 50%;
left: 50%;
width: 20px;
height: 20px;
margin-top: -10px;
margin-left: -10px;
border-radius: 50%;
border: 2px solid #ffffff;
border-top-color: #000000;
animation: spinner 2s linear infinite;
}
Adding the Button
Now that we have our styling in place, let’s add our button to the HTML:
<button mat-raised-button color="primary" [class.spinner]="loading" [disabled]="loading" (click)="createPost()">
{{ buttonText }}
</button>
Your IDE will now complain about the function createPost
and variable loading
not existing, so that’s what we’ll fix in the next step!
Finishing Up Our Button Loader
Here’s the function we’ll use to simulate a post being uploaded (BTW, great example of when to use a loading spinner inside a button):
loading = false;
buttonText = "Create Post";
createPost() {
this.loading = true;
timer(2000).subscribe(() => {
this.buttonText = "Post Created";
this.loading = false;
});
}
Above the function are our variables to keep track of the loader and show the button text
Result
If you followed this tutorial, your component should now look like this:
import { Component } from "@angular/core";
import { timer } from "rxjs";
@Component({
selector: "loader-button",
template: `
<button mat-raised-button color="primary" [class.spinner]="loading" [disabled]="loading" (click)="createPost()">
{{ buttonText }}
</button>
`,
styles: [
`
@keyframes spinner {
to {
transform: rotate(360deg);
}
}
.spinner:before {
content: "";
box-sizing: border-box;
position: absolute;
top: 50%;
left: 50%;
width: 20px;
height: 20px;
margin-top: -10px;
margin-left: -10px;
border-radius: 50%;
border: 2px solid #ffffff;
border-top-color: #000000;
animation: spinner 2s linear infinite;
}
`,
],
})
export class LoaderButtonComponent {
loading = false;
buttonText = "Create Post";
createPost() {
this.loading = true;
timer(2000).subscribe(() => {
this.buttonText = "Post Created";
this.loading = false;
});
}
}
To test it out you can either add a route to your component or add the component selector to your app component’s HTML. I added it to my app component, like this:
<loader-button/>
Then, just run your application with npm start
and you should get the following result:
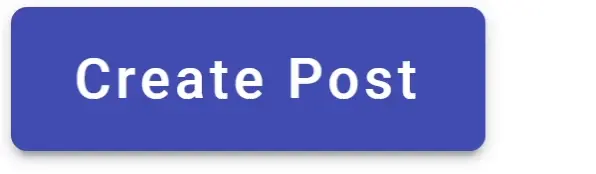
That’s all there’s to it! Pretty sweet, eh?
Conclusion
In this quick tutorial, we’ve covered the three steps to add loading spinners to Angular Material buttons. In a couple of minutes and with just some lines of code, you improved the functionality of the regular mat-button
in Angular Material.
Speaking of loading spinners… We have a great tutorial on how to add a custom color to the Angular Material mat-spinner
, check out the tutorial here.
BIG NEWS: thereās a brand new software being launched today that legally tricks AI chatbots into recommending YOUR website.
Go check it out here ==>> https://ext-opp.com/ProfitSGE
Thatās right: Just imagineā¦thereās 1.5 billion people using AI chatbots every day.
What if every time someoneā¦
-> Searched for ābest laptops for my needsā⦠the AI would show them your website?
-> Asked ChatGPT for ābest doctors in my cityā⦠it would send them to your local clientās business?
-> Begged Google Gemini for āFAST weight lossā⦠you guessed it, Gemini would FORCE them to visit your site, display your affiliate offer and fill your pockets full of sales!
This is a TRAFFIC & SEO revolution unlike anything thatās ever come before.
This is YOUR chance to legally āhijackā traffic from 1.5 billion AI chatbots users and funnel it straight to any offer, site, product ā for yourself or your clients!
Get your copy here ==>> https://ext-opp.com/ProfitSGE