The async pipe is the fastest and easiest way to handle subscriptions of observables in your Angular application.
When you create or destroy a component, the async pipe automatically subscribes to and unsubscribes from observables and promises. This helps stop memory leaks and simplifies working with async data in your templates.
In this article, we’ll look at the async pipe in Angular and some examples of how to use it.
What Is AsyncPipe in Angular?
When developing frontend applications in Angular it is almost certain you will need to fetch data from some backend. This is often accomplished by making an HTTP request to an API. The result you receive from the API will be handled in Angular using Observables
and then subscribed to using the async pipe. (illustrated below):
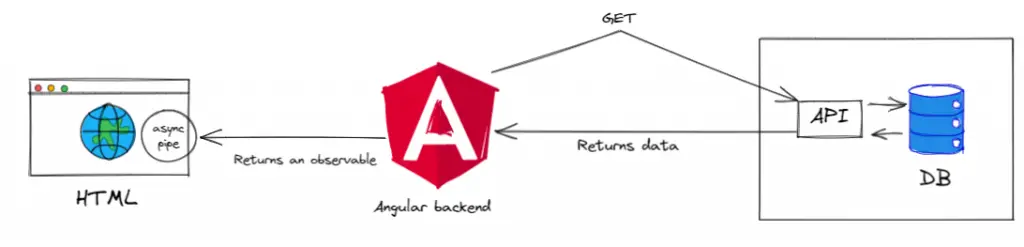
The async pipe in Angular makes subscription and handling of observables and promises much easier. It provides automatic handling (subscribing, unsubscribing) of observables and promises.
Benefits of using the async pipe:
- Reduce the risk of memory leaks
- Easy to implement
- Handles subscriptions automatically
How to Use Async Pipes in Angular
It is easy to use the async pipe in Angular. Let’s say you have this function in your application:
public get(url: string): Observable<string> {
return this.http.get<string>(url);
}
You could then display the data in your application by simply calling the function and subscribing to the returned Observable
using an async pipe:
<p>{{(httpService.get('https://catfact.ninja/fact') | async).fact}}</p>
This results in the following in our frontend:
A cat can jump up to five times its own height in a single bound
Handling JSON Objects with Async Pipes
The most common type of data structure returned from APIs and services today is the JSON (JavaScript Object Notation). The async pipe in Angular is capable of handling observables returning JSON objects.
Let’s say you have a webpage where you want to display information about a user and you have a function, called getUserInfo()
, which returns this JSON object:
{
"username": "BullishVince",
"name": "Vincent",
"last_online": "Sun Dec 25 2022 23:36:10 GMT+0100",
"registration_date": "Mon Oct 17 2022 16:12:09 GMT+0100"
}
You could display this data in a nice way on your webpage using async pipes:
<ng-container *ngIf="getUserInfo() | async as userInfo">
Username: {{userInfo.username}}<br>
Name: {{userInfo.name}}<br>
Last seen: {{userInfo.last_online}}<br>
Registered at: {{userInfo.registration_date}}<br>
</ng-container>
Conclusion
The async pipe in Angular is a useful tool when working with observables and promises. Async pipes make it easy to use observables and promises by automatically subscribing and unsubscribing, thus reducing the risk of memory leaks.
I would recommend using async pipes if you need to display the data on the front end. You can learn more about Angular async pipes here
Hope you learned new stuff from this article😉If you are interested in learning more about Angular, check out our other articles over here