Angular directives are classes that can change the appearance or behavior of elements loaded into the DOM (Document Object Model).
An Angular custom directive is used to modify the DOM to achieve your application’s specific appearance or behavior, based on your needs.
These types of directives exist in Angular:
Since custom directives are not built-in directives, we will only discuss attribute- and structural directives in this article.
What Is a Directive in Angular
As we’ve already said in the introduction, directives are classes in Angular that can change the DOM of your website, either the appearance or the behavior of the elements loaded for the user.
What Is Attribute Directives in Angular
Attribute directives modify the appearance or behavior of existing DOM elements.
For example, maybe you want to highlight elements with a red color. This can easily be accomplished with a custom attribute directive. Let’s do that!
What Are Structural Directives in Angular
Structural directives in Angular are similar to attribute directives. The main difference between these two is that the structural directive adds or removes elements inside the DOM, while attribute directives modify them.
Going forward, we’ll show you an example of how to create an Angular custom directive, in this example, a custom attribute directive.
Creating Your First Angular Custom Directive
Let’s start by generating a new Angular directive, using the Angular CLI in your terminal (I use Bash):
ng g d highlight
Then change the selector to highlight
and update the constructor to this:
constructor(private element: ElementRef) {
this.element.nativeElement.style.backgroundColor = 'red';
}
Your highlight directive should now look like this:
import { Directive, ElementRef } from '@angular/core';
@Directive({
selector: '[highlight]'
})
export class HighlightDirective {
constructor(private element: ElementRef) {
this.element.nativeElement.style.backgroundColor = 'red';
}
}
highlight.directive.tsNow you can highlight HTML elements with red using this directive:
<h1 highlight>BullishLife.com</h1>
app.component.htmlResults
Now, run your application and navigate to it using your web browser. The result looks like this:
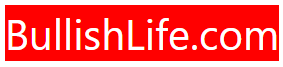
Conclusion
As you have seen, creating custom directives in Angular is easy and fast.
Note that this was just a basic example to get you started working with custom directives. By utilizing Angular custom directives, you can create much more advanced features than the example shown in this tutorial.
If you liked this short tutorial about custom directives in Angular, then you should check out our other Angular tutorials over here.