Typescript and Javascript are today the most popular web development languages. This article will go through the differences between the two programming languages and explain the strength of both depending on your requirements and use case.
Without further ado, let’s start by explaining what Javascript and Typescript are.
What Is Javascript?
Javascript is a programming language that allows you to mainly add logic to web pages. Why I say mainly is because that’s what the original purpose of the Javascript programming language was. Nowadays, you can also use Javascript for backend applications, with the help of frameworks and environments such as NodeJs.
What Is Typescript?
Typescript is a superset of the programming language Javascript. In layman’s terms, this basically means that Typescript is built upon Javascript but with more features aimed toward readability and maintenance, as opposed to how some Javascript code may look like.
Here’s an example of short code snippets, first written using Javascript and then written using Typescript. Look at both code snippets and see for yourself how code may look more readable and maintainable in Typescript than in Javascript.
// JavaScript Code
function getName({name, age}) {
return `Hi ${name}, you are ${age} years!`
}
console.log(getName({name: 'Vincent', age: 28}))
console.log(getName({name: 'Alice', age: true}))
JavaScriptWhat this function does is basically take in a name and age as parameters and then return the string Hi ${person.name}, you are ${person.age} years!
Here’s what it looks like in the console when we run our Javascript code:
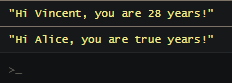
Now, here’s the same function written in Typescript:
// TypeScript Code
interface Person {
name: string;
age: number;
}
function getName(person: Person): Person {
return `Hi ${person.name}, you are ${person.age} years!`;
}
person: Person = {name: "Vincent", age: 28};
console.log(getName(person))
console.log(getName({ name: "Alice", age: true }));
TypeScriptHere’s what the console output looks like when we run our Typescript code:

The first call to getName
is valid but the second one does not send in an object of the type Person
into the function. This is a good thing about Typescript since this can help detect errors before you even think about releasing your application.
There are also a couple of improvements here compared to the code snippet written in Javascript.
The first improvement here is that the parameters used in the getName
function are typed. This means that the Typescript transpiler will make sure that only string parameters can be sent in as names and only numeric parameters can be sent in as an age.
The second improvement is that we can define the input parameter structure as an interface, thus improving the maintainability and making it easier to follow an application contract.
There are more benefits to using Typescript than the two I mentioned above. If you’re interested in learning more about the benefits of using Typescript over Javascript, check out this article which covers it pretty well.
Is Typescript Better Than Javascript?
Typescript wasn’t invented with the intention of ever replacing Javascript. Instead, Typescript complements Javascript.
There’s no real answer to whether or not Typescript is better than Javascript. You need to test both programming languages and see for yourself.
Should I Skip Javascript and Learn Typescript?
Same as the question above (Is Typescript Better Than Javascript?), there’s also no real answer to this question.
If I were to give my opinion on this, I would say that you can learn Typescript without knowing anything about Javascript. Since Typescript is built upon Javascript (and therefore has all the functionality featured with Javascript) and offers more extensive features towards increasing maintainability, it could be a great experience to start learning Typescript without previous experience in Javascript, you will most certainly also learn Javascript throughout your learning.
Conclusion
In conclusion, it is clear that Typescript offers more features for improving the readability and maintainability of your code. Even though it may seem that Typescript is a replacement for Javascript, it will not replace Javascript but instead, complement it.
If you’re just getting started getting into web development and are thinking about whether or not you should start by learning Javascript or Typescript, hopefully, this article helped in making up your mind.
Do you know that we also offer a bunch of great tutorials on how to improve the user experience and elegance of your Angular applications with the help of Angular Material? You can find a list of all our Angular Material tutorials here!